Dash and Django are two Python frameworks that can be used to build websites and web apps. But what makes them different? And which one should you pick for your projects?
You will find in this article a short description of both frameworks and two code examples of uses cases that suit the former and the latter. At the end, we summarize the comparison for Dash vs. Django in comprehensive tables. Let’s dive in!
TL;DR. While Django is a perfect framework for traditional websites, Dash shines in creating interactive web applications easily. The choice depends on what you need to build and if you want 100% Python or not.
About Django
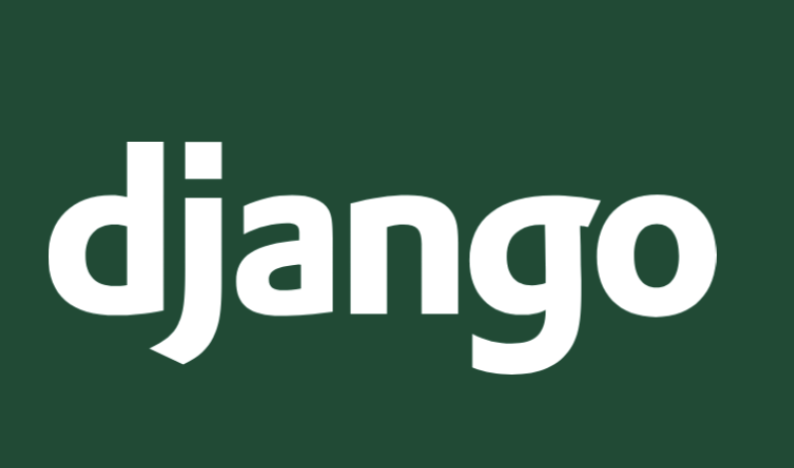
Django is a full-featured web framework that’s designed for building complex web applications. It excels at:
- Building traditional web applications with user authentication, database management, and admin interfaces
- Content management systems and e-commerce platforms
- RESTful API development
- Handling complex database operations with its powerful ORM
- Managing user sessions, forms, and security features
Example: Django is an excellent solution if you’re building an e-commerce website that needs user registration, product management, shopping carts, and payment processing.
It is also noteworthy that Django has a strong extension ecosystem and large community.
About Dash
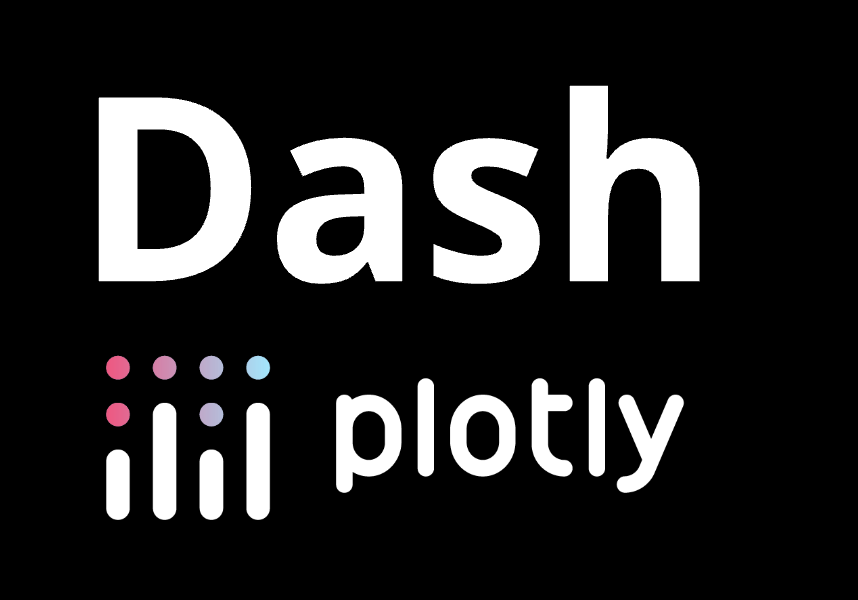
Dash Plotly, on the other hand, is specifically designed for building analytical web applications and data visualization dashboards. Its key strengths are:
- Creating interactive web applications 100% in Python
- Real-time data updates and callbacks
- Deep integration with Plotly’s visualization library
- Building data-heavy applications for data scientists and analysts
- Rapid prototyping of data visualization interfaces
Example : Dash is an excellent choice if you’re providing a web application with data visualization that requires a lot of interactivity, such as a monitoring & analysis platform.
Dash is a growing framework with a vibrant community. The recent framework updates tend to fill the gap for more classic website features (e.g. built-in URL routing was added in 2022).
Code comparison
I assume that if you read this, you are somehow familiar with Python. So let’s take a look at some tangible examples, with code. That will be much more illustrative.
Where Dash is better
The most distinctive built-in feature of Dash is its reactive callback system for building interactive data visualizations without JavaScript.
Here is a code example for Dash:
from dash import Dash, dcc, html, Input, Output
import plotly.express as px
app = Dash(__name__)
app.layout = html.Div([
dcc.Dropdown(
id='category-dropdown',
options=[{'label': x, 'value': x} for x in ['A', 'B', 'C']],
value='A'
),
dcc.Graph(id='interactive-graph')
])
@app.callback(
Output('interactive-graph', 'figure'),
Input('category-dropdown', 'value')
)
def update_graph(selected_category):
# This automatically re-renders when dropdown changes
df = get_data(selected_category)
return px.scatter(df, x='x', y='y')
The code illustrates how straightforward it is. First we define the app layout containing a graph, then this graph is updated with a callback function (update_graph
). That’s it!
It does not require any JavaScript code, everything is handled in Python.
To achieve the same in Django, we would need to build an API endpoint and use JavaScript to get the data, then plot the data. Let’s see the code:
# urls.py
urlpatterns = [
path('', views.dashboard, name='dashboard'),
path('api/data/<str:category>', views.get_graph_data, name='get_graph_data'),
]
# views.py
def dashboard(request):
return render(request, 'dashboard.html', {
'categories': ['A', 'B', 'C']
})
def get_graph_data(request, category):
df = get_data(category)
return JsonResponse({
'x': df['x'].tolist(),
'y': df['y'].tolist()
})
# dashboard.html
{% block content %}
<select id="category-dropdown">
{% for category in categories %}
<option value="{{ category }}">{{ category }}</option>
{% endfor %}
</select>
<div id="graph"></div>
<script>
// Need to write custom JavaScript for interactivity
document.getElementById('category-dropdown').addEventListener('change', function(e) {
fetch(`/api/data/${e.target.value}`)
.then(response => response.json())
.then(data => {
Plotly.newPlot('graph', [{
x: data.x,
y: data.y,
type: 'scatter'
}]);
});
});
</script>
{% endblock %}
In Django, every interaction must be handcrafted (in vanilla JS or with a framework such as React or Angular). Dash provides it “out-of-the-box” and as well be paired with JavaScript or extended with React components if needed.
Where Django is better
Even if we can handle user login with Dash and flask-login, the out-of-the-box authentication is really basic. On the other hand, Django automatically handles CSRF protection, password hashing, and session security.
It also provides built-in protection against common vulnerabilities (XSS, CSRF, SQL injection) :
# DJANGO - Secure by default
# views.py
from django.contrib.auth.forms import UserCreationForm
from django.shortcuts import render, redirect
def register(request):
if request.method == 'POST':
form = UserCreationForm(request.POST)
if form.is_valid():
form.save() # Handles password hashing, validation
return redirect('login')
return render(request, 'register.html', {'form': form})
# template
<form method="post">
{% csrf_token %} # CSRF protection automatic
{{ form.as_p }} # XSS protection automatic
<button type="submit">Register</button>
</form>
With Dash, you need to implement these security features yourself (at the risk of not doing them correctly):
# DASH/FLASK - Manual security setup
from dash import Dash, html, dcc, Input, Output, State
from werkzeug.security import generate_password_hash
import secrets
app = Dash(__name__)
app.server.secret_key = secrets.token_hex(16)
app.layout = html.Div([
# Must manually add CSRF token
html.Div(id='csrf-token', style={'display': 'none'},
children=secrets.token_hex(16)),
dcc.Input(id='username', type='text'),
dcc.Input(id='password', type='password'),
html.Button('Register', id='register-button'),
])
@app.callback(
Output('output', 'children'),
Input('register-button', 'n_clicks'),
State('username', 'value'),
State('password', 'value'),
State('csrf-token', 'children')
)
def register(n_clicks, username, password, csrf_token):
if n_clicks:
# Must manually implement:
# - CSRF validation
# - Input sanitization (XSS protection)
# - Password validation
hashed = generate_password_hash(password)
# Manual database insertion with SQL injection protection
In that case, working with Django provides a safer and more stable base.
Dash plotly vs. Django: table comparison
We compared each framework feature regarding how it is either built-in (✅), possible with extension (—), or needs to be implemented manually (❌).
Web Application Features
Feature | Django | Dash | Notes |
---|---|---|---|
Admin Interface | ✅ | ❌ | Django’s auto-admin is unique |
Database ORM | ✅ | — | Django ORM vs SQLAlchemy/SQL |
Authentication | ✅ | ❌ | Built-in vs Flask-Login |
Template Engine | ✅ | ❌ | Django templates vs React |
URL routing | ✅ | ✅ | Django routing is much more scalable |
Internationalization (i18n) | ✅ | ❌ | Django has built-in i18n tools |
Django shines in traditional web development with its comprehensive built-in features. The admin interface is particularly powerful, automatically generating a CRUD interface for your models. While Dash (through Flask) can achieve similar functionality, it requires additional setup and packages.
Data Visualization & Interactivity
Feature | Django | Dash | Notes |
---|---|---|---|
Interactive Graphs | — | ✅ | Dash: deep Plotly integration |
Real-time Updates | — | ✅ | Dash: built-in reactivity |
Callback System | ❌ | ✅ | Dash’s core feature |
Component State | — | ✅ | Dash: automatic state management |
This is where Dash truly excels. Its reactive callback system allows you to build complex interactive visualizations without writing JavaScript. Components automatically update when their inputs change, making it easier and faster to build interactive web apps.
Development & Deployment
Django provides a more mature development environment with comprehensive testing tools and database migration systems. Dash, while having a smaller ecosystem, integrates seamlessly with data science tools like pandas and numpy.
Feature | Django | Dash | Notes |
---|---|---|---|
Testing Framework | ✅ | ✅ | |
Database Migrations | ✅ | ❌ | Django: built-in |
Community | ✅ | ✅ | Django has larger ecosystem |
Data Science Tools | — | ✅ | Better integration with pandas/numpy |
Both frameworks can be deployed similarly, but Django offers more out-of-the-box deployment options and production-ready features. That’s because Django benefits from a more mature ecosystem and developer community.
Conclusion
As you may have understood, the difference between these two Python frameworks isn’t about their capability— they both provide the ability to develop any web application. It is more about how “built-in” certain features are.
At the end, the choice depends on 1) the type of website or web app you need to build and 2) your skill set:
- Choose Django if you need a stable framework to build a traditional website or if you have JavaScript knowledge to handle the interactivity.
- Choose Dash if you need to build an interactive web app that embeds some data visualization and/or if you only know Python.
I hope this article helped you better understand the differences between Django and Dash, just as it helped me!
You might also be interested by…
- Build a To-Do app in Python with Dash (part 2/3)In part 1, we built a basic To-Do application with Dash and Dash Mantine Components (DMC). We created a single […]
- Build a To-Do app in Python with Dash (part 1/3)In this tutorial, we’ll build a To-Do application 100% in Python using Dash plotly and the community extension Dash Mantine […]
- Dash callbacks best practices (with examples)As a Dash app grows, it is easy to get lost in the complexity of interconnected, large callbacks handling a […]
- Tutorial: Dash callbacks (schemas & examples)At its core, Dash Plotly uses a system of “callbacks” to create interactive features – these are Python functions that […]
- Dash plotly vs. Django: what are the differences?Dash and Django are two Python frameworks that can be used to build websites and web apps. But what makes […]